Using Entra authentication to provide an effective SSO strategy across all application experiences enhances user experience while providing a high level of security.
In this post we'll walk through a technique that leverages Entra Authentication using the MSAL OAuth library.
Overview
To complete the Entra + Streamlit integration, we'll implement the following steps:
- Create an App Registration in Entra
- Install the streamlit-msal dependency
- Configure the MSAL client ID and Tenant ID
- Provide a UI to trigger a login operation
Video Walk-Through
A 6-minute video walk through of this process is available on YouTube:
YouTube Walk-Through
Install Dependency
To integrate with Entra via MSAL, we'll leverage an open-source package published by Wilian Zilv. This package implements the MSAL integration using an embedded React codebase that itself leverages the Microsoft MSAL library for Javascript.
For reference, here's a link to Wilian's package on GitHub:
We'll integrate this package by adding it to our basic Streamlit app's requirements.txt
:
streamlit
streamlit-msal
Create an App Registration
The streamlit-msal
dependency will need the Client ID and Tenant ID from an app registration.
The app registration should be configured with the following elements:
Setting | Value |
---|---|
App Name | The descriptive app name that the user will see when consenting to app permissions |
Account Types | This directory only |
Redirect URI | https://localhost:8501 (for local development) |
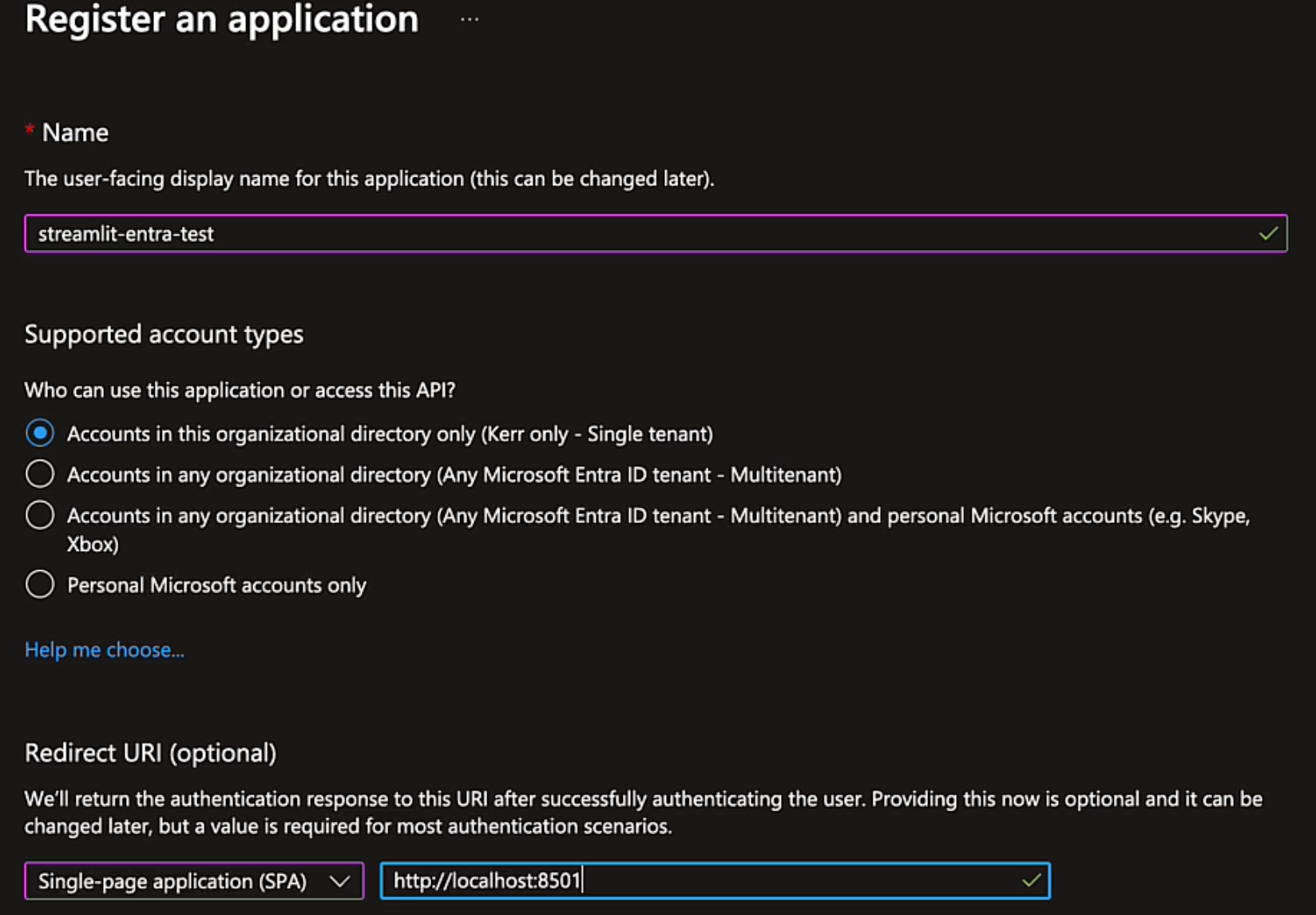
Save Application Registration IDs
Save the Client ID and Tenant ID from the App Registration Overview screen. These values will be added to the Streamlit application in the next step.
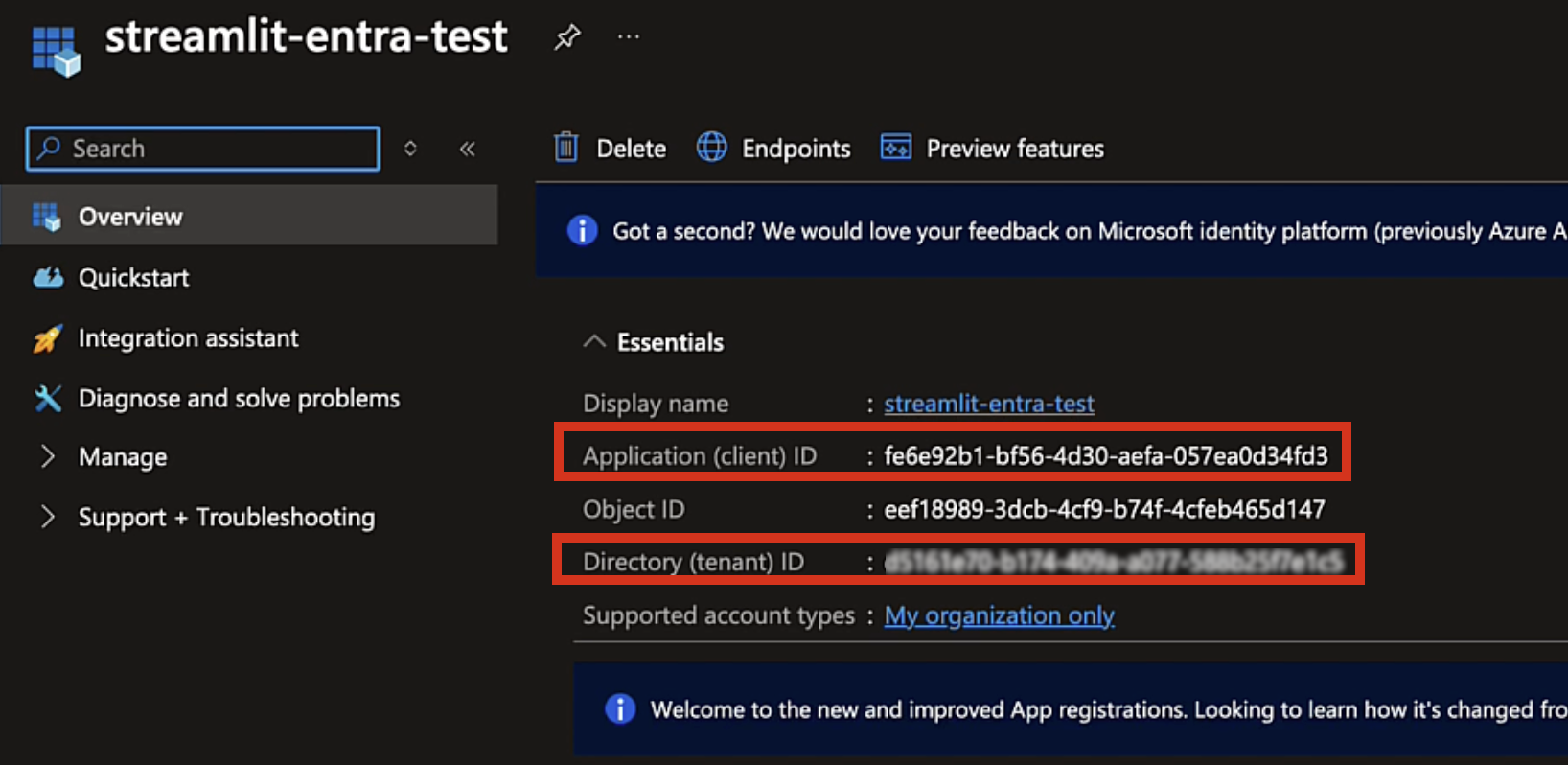
Add Imports to app.py
Begin the Streamlit app by adding imports to the top of the app.py
file.
import streamlit as st
from streamlit_msal import Msal
Add MSAL configuration
The streamlit-msal
library needs the Client ID, Tenant ID, Authority, and desired scope(s) to request. We configure this by intializing streamlit-msal
with these values.
auth_data = Msal.initialize(
client_id=f"{client_id}",
authority=f"https://login.microsoftonline.com/{tenant_id}",
scopes=["User.Read"], # Ask for a basic profile user info claim
)
Add login to the UI
In this sample app, we'll provide a button that begins the OAuth flow between Streamlit and Entra.
if st.button("Sign in"):
Msal.sign_in() # Show popup to select account
Use the result
After a successful login, the streamlit-msal
library will update the access_token
variable with the Entra token as a JSON object (technically a JSON Web Token or JWT).
access_token = auth_data["accessToken"]
st.json(auth_data)
Error Handling
This post is kept as simple as possible to illustrate the bare minimum coding to implement Entra Auth with a Streamit app. This sample doesn't account for errors and edge cases, which a production app should take into account--so consider this an introductory primer!
Full Streamlit App Code
import streamlit as st
from streamlit_msal import Msal
# For a deployed app, place these variables in an environment variable
client_id = "Client ID of Entra App Registration"
tenant_id = "Tenant ID of Entra App Registration"
# Initialize the Msal object
auth_data = Msal.initialize(
client_id=f"{client_id}",
authority=f"https://login.microsoftonline.com/{tenant_id}",
scopes=["User.Read"],
)
if st.button("Sign in"):
Msal.sign_in() # Show popup to select account
if not auth_data:
st.write("You are not signed in")
else:
access_token = auth_data["accessToken"]
st.json(auth_data)
Github Repo Link
The project used in this post is available on my GitHub repo: